How to Use BleuIO as a USB Serial Device on Android with Capacitor
March 11, 2025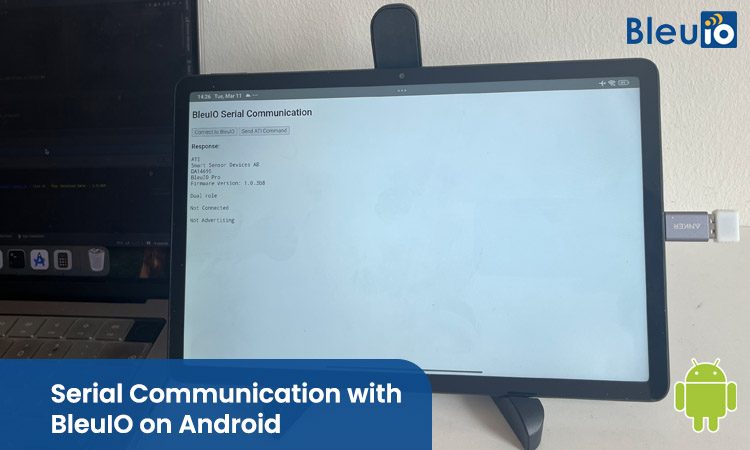
This project demonstrates how to use the BleuIO USB dongle as a serial port on an Android device via OTG (On-The-Go) USB. With Capacitor 6 and the @adeunis/capacitor-serial
plugin, we establish a serial connection, send AT commands, and read responses in real time. This project works with both BleuIO and BleuIO Pro.
This tutorial provides a step-by-step guide to setting up serial communication with BleuIO on Android. The project connects to BleuIO via a serial port, sends basic ATI commands, and displays the received response on the screen. This example serves as a starting point for creating, testing, and debugging Bluetooth Low Energy (BLE) applications. It can be expanded to support additional commands and functionalities based on specific needs. The full source code is available for customization and further development.
Use Cases
The BleuIO USB dongle can be used in various applications that require serial communication on Android. One of the most common applications is Bluetooth Low Energy (BLE) development, where developers need to configure and test BLE modules by sending and receiving AT commands. This project allows for mobile debugging and real-time configuration of BLE devices without needing a PC.
Additionally, this setup is valuable for IoT and embedded systems, where devices communicate over a serial connection. With an Android phone acting as a serial terminal, engineers and developers can test, monitor, and debug hardware components in the field without requiring a laptop. Another important use case is USB-to-serial debugging, where embedded system engineers need to send commands and receive logs directly from an Android device using OTG.
For those working with sensor modules, microcontrollers, or custom embedded systems, this project simplifies the process of sending commands and reading responses directly from a mobile device. It also serves as an excellent starting point for developing Android applications that require serial communication through Capacitor.
Why Do We Need This?
Android devices do not natively support USB-to-serial communication. Unlike computers, which come with built-in serial drivers and terminal software, Android does not provide a direct way to communicate with serial devices over USB. This makes it difficult for developers, engineers, and embedded system designers to interact with BLE modules, sensors, and microcontrollers.
By using Capacitor 6 with the @adeunis/capacitor-serial
plugin, we can bridge this gap and allow Android devices to function as serial terminals. This is especially useful when working with devices like BleuIO, where real-time communication is essential for configuring, testing, and debugging Bluetooth applications.
This project removes the need for external adapters or complex Android development, leveraging Capacitor’s web-based approach. It offers a simple and scalable way to integrate serial communication into mobile applications without requiring in-depth knowledge of Android’s USB APIs or native development tools.
Requirements
To use this project, you need a few essential components to establish serial communication between an Android device and BleuIO.
- BleuIO or BleuIO Pro – The USB dongle used for Bluetooth Low Energy (BLE) communication. This project is designed to interact with BleuIO over a USB serial connection.
- Android Device – A smartphone or tablet that supports USB OTG (On-The-Go), allowing it to function as a USB host and communicate with external devices.
- OTG Cable or Adapter – Required to connect BleuIO to the Android device. Since most smartphones have USB-C or Micro-USB ports, an OTG adapter is needed to interface with the USB-A connector of BleuIO.
Installation & Setup
Install Node.js & Capacitor 6
BleuIO communication requires the @adeunis/capacitor-serial plugin, which is only compatible with Capacitor 6.
👉 First, install Node.js (if not installed):
Download Node.js
Create a Capacitor 6 Project
mkdir bleuio-serial
cd bleuio-serial
npm init -y
npm install @capacitor/core@6 @capacitor/cli@6
Install Android Platform
npm install @capacitor/android@6
npx cap add android
Install Serial Communication Plugin
npm install @adeunis/capacitor-serial
Ensure JDK 17 is Installed
Capacitor 6 requires JDK 17. Install it via:
sudo apt install openjdk-17-jdk # Linux
brew install openjdk@17 # macOS
Verify installation:
java -version
It should output something like:
openjdk version "17.0.x"
OTG Permissions on Android
Why Do We Need OTG Permissions?
- Android devices do not natively support serial USB communication.
- OTG permissions allow USB host mode, so Android can communicate with external serial devices.
How to Enable OTG Permissions?
Modify AndroidManifest.xml
:
<uses-feature android:name="android.hardware.usb.host"/>
<uses-permission android:name="android.permission.USB_PERMISSION"/>
Then, create device_filter.xml
inside android/app/src/main/res/xml/
:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<usb-device vendor-id="0x2DCF" product-id="0x6002" />
</resources>
These values match the BleuIO Vendor ID & Product ID, allowing the system to recognize it.
Project Code Explanation
index.html
This file provides buttons to connect to BleuIO and send AT commands.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>BleuIO Serial Communication</title>
</head>
<body>
<h2>BleuIO Serial Communication</h2>
<button onclick="connectToSerial()">Connect to BleuIO</button>
<button onclick="sendATCommand()">Send ATI Command</button>
<p><strong>Response:</strong></p>
<pre id="response">Waiting for response...</pre>
<script src="index.js" defer></script>
</body>
</html>
index.js
This file:
- Requests USB permission
- Opens a serial connection
- Sends and reads AT commands
- Handles continuous data streaming from BleuIO
const Serial = window.Capacitor.Plugins.Serial;
// Vendor ID & Product ID for BleuIO
const VENDOR_ID = 0x2DCF;
const PRODUCT_ID = 0x6002;
let fullResponse = ""; // Stores incoming data
async function connectToSerial() {
console.log("Requesting permission for BleuIO...");
try {
const permissionResponse = await Serial.requestSerialPermissions({
vendorId: VENDOR_ID,
productId: PRODUCT_ID,
driver: "CDC_ACM_SERIAL_DRIVER"
});
if (!permissionResponse.granted) {
console.error("Permission denied!");
document.getElementById("response").textContent = "Permission denied!";
return;
}
console.log("Opening serial connection...");
await Serial.openConnection({
baudRate: 115200,
dataBits: 8,
stopBits: 1,
parity: 0,
dtr: true,
rts: true
});
console.log("Serial connection opened!");
document.getElementById("response").textContent = "Connected to BleuIO!";
fullResponse = ""; // Clear previous response
// Register read callback to receive data continuously
await Serial.registerReadCallback((message, error) => {
if (message && message.data) {
console.log("Received Data:", message.data);
fullResponse += message.data + "\n";
document.getElementById("response").textContent = fullResponse;
} else if (error) {
console.error("Read error:", error);
}
});
} catch (error) {
console.error("Connection error:", error);
document.getElementById("response").textContent = "Connection error.";
}
}
async function sendATCommand() {
console.log("Sending ATI command...");
try {
fullResponse = ""; // Clear response buffer
await Serial.write({ data: "ATI\r\n" });
console.log("ATI command sent!");
console.log("Waiting for response...");
} catch (error) {
console.error("Command error:", error);
}
}
// Expose functions globally
window.connectToSerial = connectToSerial;
window.sendATCommand = sendATCommand;
Running the App
Sync and Build
npx cap sync android
npx cap run android
Open the App
- Connect BleuIO to Android via OTG.
- Click “Connect to BleuIO” → A permission prompt should appear.
- Click “Send ATI Command” → Response should appear.
Final Results
After running, you should see output like:
Smart Sensor Devices AB
DA14695
BleuIO Pro
Firmware Version: 1.0.3b8
Dual role
Not Connected
Not Advertising
Screenshots
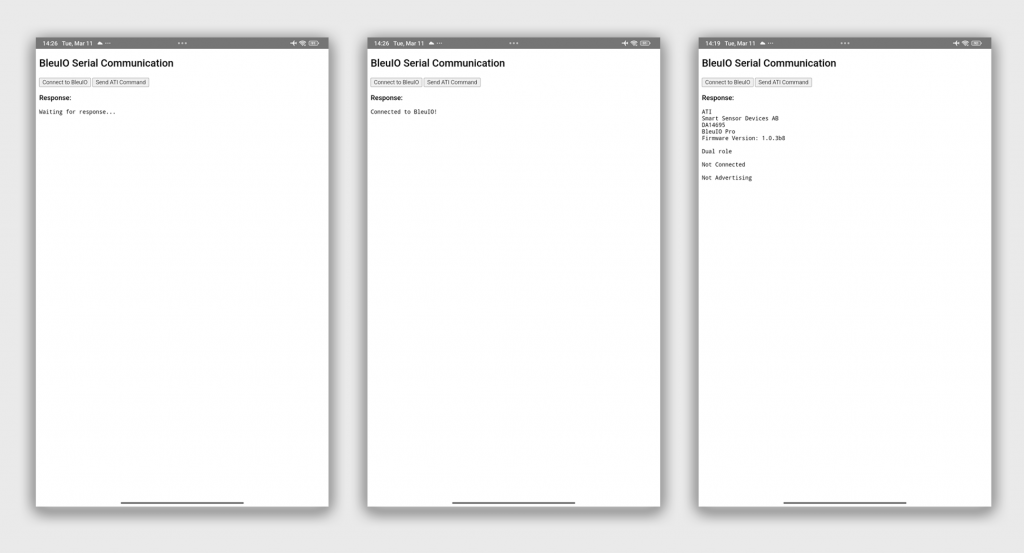
Source code
https://github.com/smart-sensor-devices-ab/bleuio-serial-android
This project demonstrates how to use the BleuIO USB dongle as a serial communication device on an Android phone. By leveraging Capacitor 6 and the @adeunis/capacitor-serial
plugin, we successfully established a serial connection, sent AT commands, and received responses via USB OTG.
With the growing demand for mobile-first development and hardware interaction via smartphones, this project provides a solid foundation for further expanding serial communication capabilities on Android.