How to Build Your Own Bluetooth Scriptable Sniffer for Under $30
April 2, 2025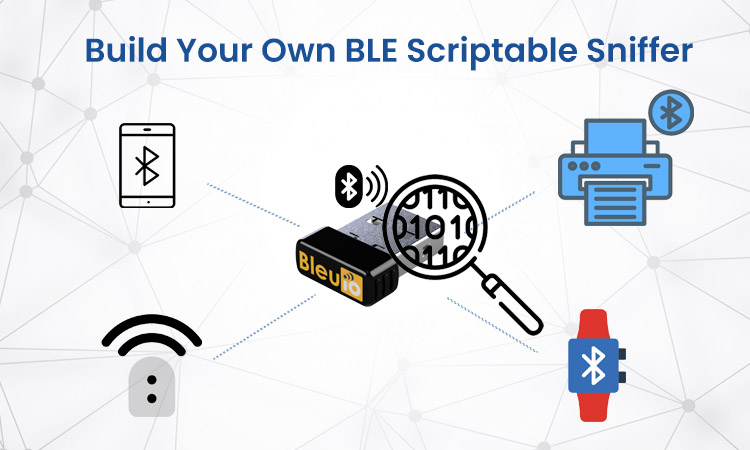
Bluetooth is commonly used in many products — from smartwatches and fitness trackers to wireless speakers, beacons, air quality sensors, and beyond. As developers, engineers, or even curious tech enthusiasts, we often would like to analyze what is being transmitted in the air interface between devices.
That’s where a Bluetooth sniffer comes into play.
What is a Bluetooth Sniffer?
A Bluetooth sniffer is a hardware or software tool that captures and monitors Bluetooth communication between devices. Think of it as a network traffic analyzer, but for Bluetooth instead of Wi-Fi or Ethernet.
It works by listening to Bluetooth advertisement packets or data exchanges, decoding them, and presenting the raw data in a human-readable format. These sniffers can help you:
- Discover nearby Bluetooth Low Energy (BLE) devices
- Monitor BLE advertisement packets
- Analyze signal strength (RSSI)
- Debug BLE applications
- Reverse engineer custom BLE services
There are high-end Bluetooth sniffers on the market — like those from Ellisys or Teledyne LeCroy — which are powerful but often cost hundreds or thousands of dollars.
But what if you could build your own for under $30?
BleuIO – BLE USB Dongle
BleuIO is a compact USB dongle that turns your computer into a Bluetooth Low Energy sniffer and data communication tool. It’s built on Dialog Semiconductor’s DA14683 chip and supports AT command communication over a virtual COM port.
You can plug it into any USB port and control it using a terminal or a script.
Price: $24.99
Platform support: Windows, macOS, Linux
Protocols supported: Bluetooth Low Energy (BLE)
Programming Language Support: Supports almost all major programming languages including C++, C#, Python, Javascript etc
Build Your Own Scriptable BLE Sniffer with BleuIO
What You’ll Need
- 1x BleuIO USB Dongle
- A computer (Windows/macOS/Linux)
- Python installed (3.x recommended)
- The
pyserial
library - The
bluetooth_numbers
library
Step 1: Install Required Python Package
Open your terminal or command prompt and run:
pip install pyserial
pip install bluetooth-numbers
Step 2: Connect the BleuIO Dongle
Plug the dongle into a USB port. On Windows, it’ll appear as something like COM3
. On macOS/Linux, it will show up as /dev/tty.usbmodemXXXX
or /dev/ttyACM0
.
Step 3: Write the BLE Sniffer Script
import serial
import time
import re
from bluetooth_numbers import company
import binascii
# Replace with your actual serial port
#SERIAL_PORT = 'COM3' # Windows
SERIAL_PORT = '/dev/cu.usbmodem4048FDE52DAF1' # For Linux/macOS
BAUD_RATE = 9600
def scan_devices(duration=3):
device_list = []
try:
with serial.Serial(SERIAL_PORT, BAUD_RATE, timeout=1) as ser:
ser.write(f'AT+DUAL\r\n'.encode())
print(f"\nStarting BLE scan for {duration} seconds...\n")
ser.write(f'AT+GAPSCAN={duration}\r\n'.encode())
time.sleep(duration + 1)
print("Discovered Devices:\n" + "-"*50)
while ser.in_waiting:
line = ser.readline().decode('utf-8', errors='ignore').strip()
print(">>", line)
match = re.match(r"\[\d+\] Device: \[(\d)\]([0-9A-F:]{17})\s+RSSI:\s*-?\d+(?:\s+\((.+?)\))?", line)
if match:
addr_type = int(match.group(1))
mac = match.group(2)
name = match.group(3) if match.group(3) else ""
device_list.append((addr_type, mac, name))
return device_list
except serial.SerialException as e:
print("Serial error:", e)
return []
def scan_target_device(mac_address, address_type=1, duration=3):
try:
with serial.Serial(SERIAL_PORT, BAUD_RATE, timeout=1) as ser:
print(f"\nScanning target device {mac_address} (Type: {address_type}) for {duration} seconds...\n")
cmd = f'AT+SCANTARGET=[{address_type}]{mac_address}={duration}\r\n'
ser.write(cmd.encode())
time.sleep(duration + 1)
print("Advertisement Data:\n" + "-"*50)
adv_data = None
while ser.in_waiting:
line = ser.readline().decode('utf-8', errors='ignore').strip()
print(">>", line)
if "Device Data [ADV]:" in line and adv_data is None:
parts = line.split("Device Data [ADV]:")
if len(parts) == 2:
adv_data = parts[1].strip()
if adv_data:
print("\nDecoding Advertisement Payload...\n")
decode_ble_adv(adv_data)
else:
print("No ADV data found to decode.")
except serial.SerialException as e:
print("Serial error:", e)
AD_TYPE_NAMES = {
0x01: "Flags",
0x02: "Incomplete 16-bit UUIDs",
0x03: "Complete 16-bit UUIDs",
0x08: "Shortened Local Name",
0x09: "Complete Local Name",
0x0A: "TX Power Level",
0x16: "Service Data",
0xFF: "Manufacturer Specific Data"
}
# Flag bit definitions
FLAGS_MAP = {
0x01: "LE Limited Discoverable Mode",
0x02: "LE General Discoverable Mode",
0x04: "BR/EDR Not Supported",
0x08: "Simultaneous LE and BR/EDR (Controller)",
0x10: "Simultaneous LE and BR/EDR (Host)"
}
def decode_ble_adv(hex_str):
data = bytearray.fromhex(hex_str)
index = 0
object_count = 1
print(f"Decoding ADV Data: {hex_str}\n{'-'*50}")
while index < len(data):
length = data[index]
if length == 0 or (index + length >= len(data)):
break
ad_type = data[index + 1]
ad_data = data[index + 2: index + 1 + length]
type_name = AD_TYPE_NAMES.get(ad_type, f"UNKNOWN")
print(f"\nData Object {object_count}:")
print(f"Length: {length}")
print(f"Type: 0x{ad_type:02X} ({type_name})")
if ad_type == 0x01: # Flags
flags = ad_data[0]
print("Flags:")
for bit, label in FLAGS_MAP.items():
if flags & bit:
print(f" - {label}")
print("Device Type Inferred:", end=" ")
if flags & 0x04:
print("LE Only")
elif flags & (0x08 | 0x10):
print("Dual Mode (LE + BR/EDR)")
else:
print("BR/EDR Only or Unknown")
elif ad_type == 0xFF: # Manufacturer Specific Data
if len(ad_data) >= 2:
company_id = ad_data[0] | (ad_data[1] << 8)
company_name = company.get(company_id, "Unknown")
print(f"Company Identifier: 0x{company_id:04X} ({company_name})")
manufacturer_data = ad_data[2:]
if manufacturer_data:
print("Manufacturer Data:", binascii.hexlify(manufacturer_data).decode())
else:
print("Malformed Manufacturer Specific Data")
elif type_name == "UNKNOWN":
print(f"This script is currently unable to decode this type.")
print("Raw Data:", "0x" + binascii.hexlify(ad_data).decode())
else:
print("Raw Data:", "0x" + binascii.hexlify(ad_data).decode())
index += length + 1
object_count += 1
if __name__ == "__main__":
devices = scan_devices()
if devices:
print("\nSelect a device to scan further:")
for idx, (addr_type, mac, name) in enumerate(devices):
label = f"{mac} ({name})" if name else mac
print(f"[{idx}] {label} ")
choice = input("Enter device number (e.g. 0): ").strip()
try:
selected = devices[int(choice)]
scan_target_device(selected[1], selected[0])
except (IndexError, ValueError):
print("Invalid selection. Exiting.")
else:
print("No devices found.")
Note: Make sure to update the
SERIAL_PORT
variable in the script to match your system’s COM port — for example,COM3
on Windows or/dev/tty.usbmodemXXXX
on macOS/Linux.
How It Works
- Step 1: Sends the
AT+GAPSCAN
command to BleuIO, which returns a list of nearby BLE devices with MAC addresses and signal strength. - Step 2: Parses the output and allows the user to select one device.
- Step 3: Sends the
AT+SCANTARGET=[address_type]MAC=3
command to scan the selected device and retrieve its advertisement payload. - Step 4: Displays the detailed advertising data broadcasted by the device — which can include device name, UUIDs, manufacturer data, and sensor readings.
- Step 5: Attempts to decode all fields in the BLE advertisement payload, showing both known fields (like Flags, Manufacturer Data) and unknown ones with raw data formatting
Decoding Advertisement Data
Every BLE advertisement is made up of TLV (Type-Length-Value) structures. This script extracts each one and attempts to decode:
- Flags (Type 0x01): e.g., LE Only, Dual Mode
- Manufacturer Specific Data (Type 0xFF): Extracts company ID and raw payload
- Unknown types: Clearly marked and printed as raw hex for future analysis
Even if the script can’t interpret a block, you’ll still see it listed with length, type, and raw content — helping with reverse engineering or debugging unknown BLE devices.
Sample Output
Starting BLE scan for 3 seconds...
Discovered Devices:
>> AT+DUAL
>> AT+GAPSCAN=3
>> SCANNING...
>> [01] Device: [1]D1:53:C9:A9:8C:D2 RSSI: -56 (HibouAIR)
>> [02] Device: [1]C4:AD:CB:84:A5:73 RSSI: -83
>> [03] Device: [1]D1:79:29:DB:CB:CC RSSI: -52 (HibouAIR)
>> [04] Device: [1]C0:8C:23:2E:1A:E5 RSSI: -59
>> SCAN COMPLETE
Select a device to scan further:
[0] D1:53:C9:A9:8C:D2 (HibouAIR)
[02] C4:AD:CB:84:A5:73
[03] D1:79:29:DB:CB:CC (HibouAIR)
[104] C0:8C:23:2E:1A:E5
Enter device number (e.g. 0): 03
Scanning target device D1:79:29:DB:CB:CC (Type: 1) for 3 seconds...
Advertisement Data:
--------------------------------------------------
>> AT+SCANTARGET=[1]D1:79:29:DB:CB:CC=3
>> SCANNING TARGET DEVICE...
>> [D1:79:29:DB:CB:CC] Device Data [ADV]: 0201061BFF5B0705042200696D009F26B60082023D00000000000000024C02
>> [D1:79:29:DB:CB:CC] Device Data [RESP]: 110750EADA308883B89F604F15F30100C98E09094869626F75414952
>> SCAN COMPLETE
Decoding Advertisement Payload...
Decoding ADV Data: 0201061BFF5B0705042200696D009F26B60082023D00000000000000024C02
--------------------------------------------------
Data Object 1:
Length: 2
Type: 0x01 (Flags)
Flags:
- LE General Discoverable Mode
- BR/EDR Not Supported
Device Type Inferred: LE Only
Data Object 2:
Length: 27
Type: 0xFF (Manufacturer Specific Data)
Company Identifier: 0x075B (Smart Sensor Devices AB)
Manufacturer Data: 05042200696d009f26b60082023d00000000000000024c02
Source Code on GitHub
You can find the full, open-source implementation of this BLE sniffer — including the Python script and all improvements — on GitHub:
https://github.com/smart-sensor-devices-ab/ble_sniffer_bleuio
Now we have a working BLE sniffer that not only scans for nearby devices but also lets you interactively select a target and read its detailed advertisement data.
Here are some cool extensions we can build from here:
- Display air quality sensor data from BLE beacons like HibouAir
- Export scan logs to CSV for analysis
- Build a desktop or web UI using Electron or Flask
- Trigger alerts based on proximity or signal strength
- Improve decoding support for more AD types (TX Power, Local Name, Services)
- Show scan response (RESP) data alongside advertisement (ADV)
- Display or log RSSI values for signal analysis
Bluetooth sniffers don’t have to be complicated or expensive. With BleuIO, a bit of Python, and a USB port, you can begin exploring the hidden world of BLE devices all around you — right from your own machine.
This setup is perfect for developers working on BLE apps, IoT product engineers, or tech enthusiasts who want to learn how devices communicate wirelessly.