Building BLE Applications with BleuIO and Go
August 7, 2024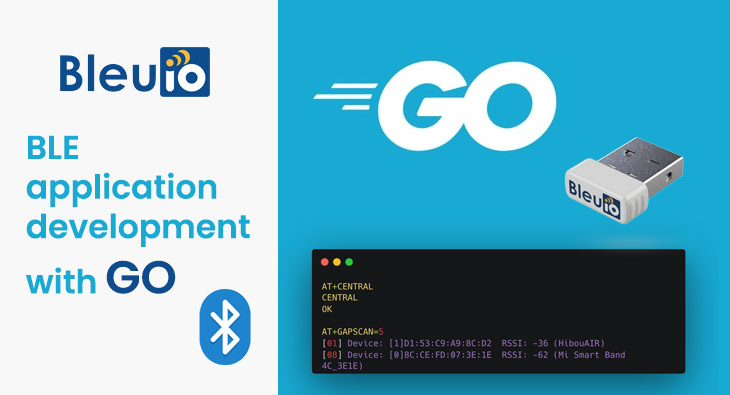
In this tutorial, we will walk you through the steps to get started with Bluetooth Low Energy (BLE) development using the BleuIO USB dongle and the Go programming language. BleuIO is a versatile and user-friendly BLE USB dongle that simplifies BLE application development with its easy-to-use AT Commands. We will show you how to set up your environment, write a simple Go program to interact with the BLE dongle, and explore some of the key features of BleuIO.
Introduction to Go
Go, also known as Golang, is an open-source programming language developed by Google. It is designed for simplicity, efficiency, and reliability, making it an excellent choice for system programming and large-scale software development. Go’s strong concurrency support, fast compilation, and robust standard library make it a popular language for network programming, cloud services, and, of course, BLE applications.
Introduction to BleuIO
BleuIO is a Bluetooth Low Energy USB dongle that can be used to create new BLE applications quickly and easily. With its built-in AT Commands, developers can interact with the BLE dongle without needing deep knowledge of BLE protocols or complex configurations. BleuIO supports various operating systems, making it a versatile tool for any development environment.
Key features of BleuIO include:
- Easy-to-use AT Commands for faster development
- Compatibility with any programming language
- Support for Windows, macOS, and Linux
Setting Up Your Development Environment
Step 1: Install Go
First, ensure that you have Go installed on your system. You can download the latest version of Go from the official website: https://golang.org/dl/. Follow the installation instructions for your operating system.
Step 2: Initialize a New Go Module
Create a directory and open a terminal . Run the following command to initialize a new Go module:
go mod init bleuio-example
Step 3: Install the Serial Package
Install the go.bug.st/serial
package, which provides a simple API for serial communication in Go:
go get go.bug.st/serial
Writing Your First Go Program with BleuIO
Step 4: Write the Program
Create a new file named main.go
in your project directory and add the following code:
package main
import (
"fmt"
"log"
"time"
"go.bug.st/serial"
)
func main() {
// Open the serial port
mode := &serial.Mode{
BaudRate: 9600,
}
port, err := serial.Open("/dev/cu.usbmodem4048FDE52CF21", mode)
if err != nil {
log.Fatalf("Failed to open port: %v", err)
}
defer port.Close()
// Write "AT+CENTRAL" to the serial port
_, err = port.Write([]byte("AT+CENTRAL\r"))
if err != nil {
log.Fatalf("Failed to write AT+CENTRAL to port: %v", err)
}
fmt.Println("Command sent: AT+CENTRAL")
// Wait for a short moment to ensure the command is processed
time.Sleep(2 * time.Second)
// Read the response for the AT+CENTRAL command
buf := make([]byte, 100)
n, err := port.Read(buf)
if err != nil {
log.Fatalf("Failed to read from port: %v", err)
}
fmt.Printf("Response from AT+CENTRAL:\n%s\n", string(buf[:n]))
// Write "AT+GAPSCAN=5" to the serial port
_, err = port.Write([]byte("AT+GAPSCAN=5\r"))
if err != nil {
log.Fatalf("Failed to write AT+GAPSCAN=5 to port: %v", err)
}
fmt.Println("Command sent: AT+GAPSCAN=5")
// Wait for the scan to complete (5 seconds in this case)
time.Sleep(6 * time.Second) // Adding a bit more time to ensure the response is received
// Read the response for the AT+GAPSCAN=5 command
buf = make([]byte, 1000)
n, err = port.Read(buf)
if err != nil {
log.Fatalf("Failed to read from port: %v", err)
}
// Print the response
fmt.Printf("Response from AT+GAPSCAN=5:\n%s\n", string(buf[:n]))
}
Step 5: Run the Program
Ensure your BleuIO USB dongle is connected and configured correctly. Then, run the program using the following command in the terminal:
go run main.go
Explanation of the Program
- Opening the Serial Port: The program opens the serial port where the BleuIO dongle is connected. Adjust the serial port path (
/dev/cu.usbmodem4048FDE52CF21
) according to your system (e.g.,COM3
on Windows). To get the location of connected BleuIO on macOS, run this command on terminal ls /dev/cu.* - Setting the Central Role: The program sends the
AT+CENTRAL
command to set the BLE dongle in central role mode. Similarly we can try sending AT+FINDSCANDATA=5B07=3 which will look for advertised data from BLE devices whose manufacturing id is 5B07. - Reading the Response: It waits for 2 seconds to ensure the command is processed and reads the response from the serial port.
- Scanning for BLE Devices: The program sends the
AT+GAPSCAN=5
command to scan for nearby BLE devices for 5 seconds. - Printing the Scan Results: After waiting for the scan to complete, the program reads and prints the response from the serial port.
Output
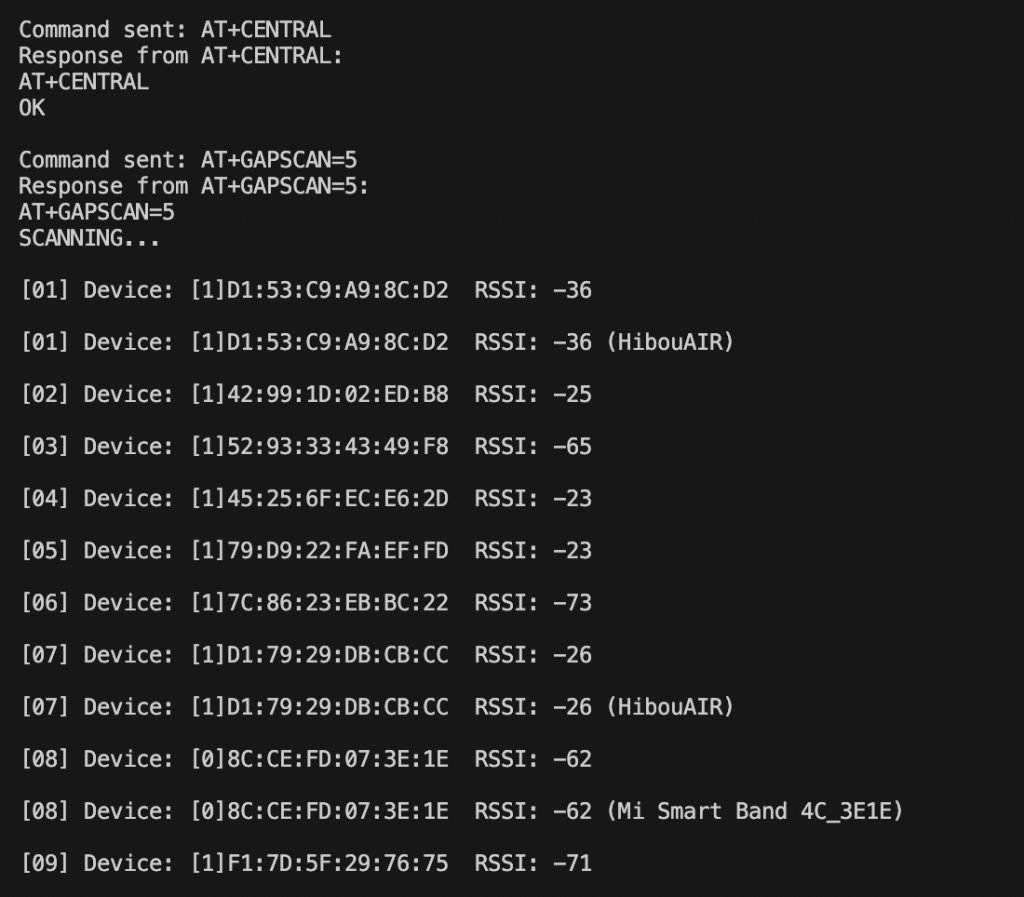
This tutorial demonstrated how to get started with BLE development using the BleuIO USB dongle and the Go programming language. BleuIO simplifies BLE application development with its straightforward AT Commands, making it accessible for developers using any programming language. With Go’s efficiency and robust standard library, you can quickly develop powerful BLE applications.